How to Convert SVG to React Component for Dynamic Styling
Posted on: Apr 18, 2025
3 mins read
Learn how to transform static SVGs into reusable React components that support dynamic styling, colour changes, and prop spreading in just a few steps.
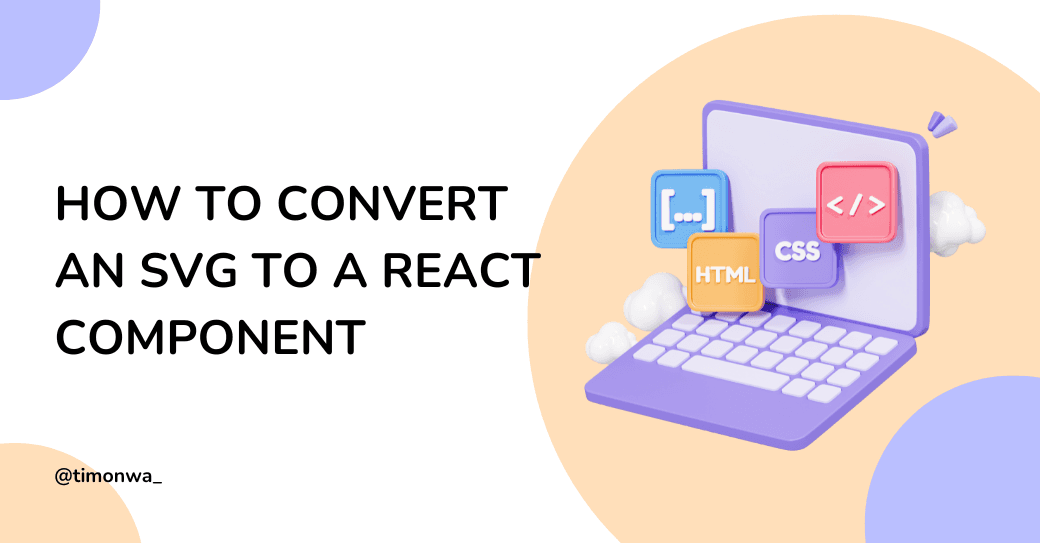
SVG to React Component
Need to use an SVG icon with dynamic styling in your React project? Rather than importing it as a static image, convert it to a React component for full control over its appearance.
The Problem
When working with SVG icons in React, you often need to:
- Change the icon's colour based on state (hover, active, etc.)
- Adjust its size in different contexts
- Apply consistent styling across your application
Importing SVGs as images or using dangerouslySetInnerHTML
limits these capabilities.
The Solution
Transform your SVG into a React component that accepts props for dynamic styling:
jsx// Before: Static SVG<svgxmlns="http://www.w3.org/2000/svg"width="24"height="24"viewBox="0 0 24 24"fill="none"stroke="black"stroke-width="2"stroke-linecap="round"stroke-linejoin="round"class="lucide lucide-menu-icon lucide-menu"><line x1="4" x2="20" y1="12" y2="12" /><line x1="4" x2="20" y1="6" y2="6" /><line x1="4" x2="20" y1="18" y2="18" /></svg>;// After: React Componentexport const MenuIcon = ({ className, ...props }) => (<svgclassName={className}xmlns="http://www.w3.org/2000/svg"width="24"height="24"viewBox="0 0 24 24"fill="none"stroke="currentColor"stroke-width="2"stroke-linecap="round"stroke-linejoin="round"{...props}><line x1="4" x2="20" y1="12" y2="12" /><line x1="4" x2="20" y1="6" y2="6" /><line x1="4" x2="20" y1="18" y2="18" /></svg>);
This approach gives you three key advantages:
- Use
className
for styling with CSS or utility classes - Replace hardcoded colours with
currentColor
to inherit parent text colour - Pass additional props with the spread operator for flexibility
Usage Example
jsxconst IconDemo = () => {return (<div className="flex items-center gap-4">{/* Basic usage */}<MenuIcon />{/* With hover effect */}<MenuIcon className="transition hover:text-blue-500" />{/* Different size and colour */}<MenuIcon className="h-14 w-14 text-green-600" /></div>);};
Figure 1: Example of SVGs converted into React components with dynamic styling.
Quick tips
- For many SVGs - Consider automating conversion with SVGR Playground
- For SVGs with multiple colours, you can add specific colour props:
jsxexport const ComplexIcon = ({className,primaryColour,secondaryColour,...props}) => (<svgclassName={className}viewBox="0 0 24 24"fill="none"xmlns="http://www.w3.org/2000/svg"{...props}><linex1="4"x2="20"y1="12"y2="12"stroke={primaryColour || "currentColor"}/><linex1="4"x2="20"y1="6"y2="6"stroke={secondaryColour || "currentColor"}/><line x1="4" x2="20" y1="18" y2="18" stroke="currentColor" /></svg>);// Usage with multiple coloursexport default function IconDemo() {return (<div className="flex items-center gap-4"><ComplexIconprimaryColour="red"secondaryColour="blue"className="h-10 w-10"/></div>);}
For a more comprehensive guide on this topic, including troubleshooting tips and advanced techniques, check out my full article: How to Convert an SVG into a React Component: A Simple Guide
Connect with Me
Did this snippet help you? I'd love to know!
Twitter • GitHub • LinkedIn • Newsletter • Buy Me a Coffee
Happy coding! 🚀